backstage/sign-in-with-github
how to set up GitHub authentication and add a user to backstage
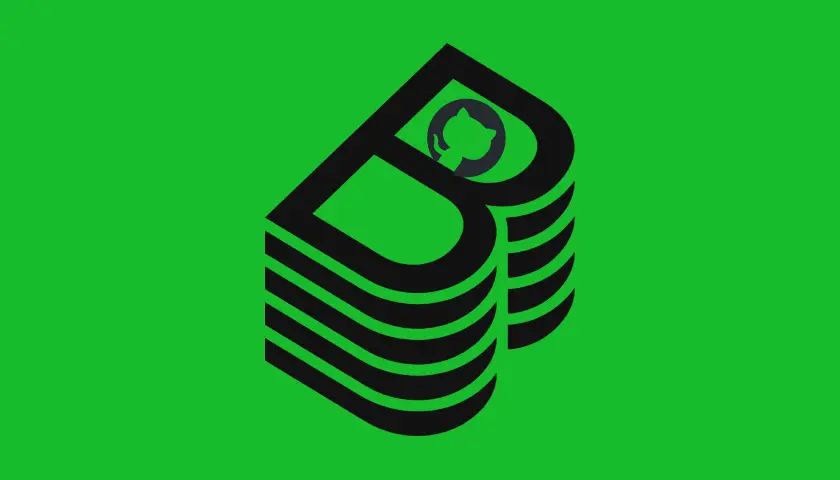
prerequisites
setup nodejs
You need to have a recent LTS version of NodeJS installed, at the time of writing that is 20. A good way to install NodeJS is using nvm, the node version manager.
Additionally, you will need yarn, which you can install using npm, which comes with node.
# install nvm
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.7/install.sh | bash
# load nvm, you should put this in your ~/.bash_profile, ~/.zshrc, ~/.profile, or ~/.bashrc
export NVM_DIR="$([ -z "${XDG_CONFIG_HOME-}" ] && printf %s "${HOME}/.nvm" || printf %s "${XDG_CONFIG_HOME}/nvm")"
[ -s "$NVM_DIR/nvm.sh" ] && \. "$NVM_DIR/nvm.sh"
# install node 20
nvm install 20
# install yarn
npm i -g yarn
initialising backstage
To get started using backstage, you will need to create a new app.
# move to the directory you would like your backstage project to be
cd ~/projects
#create backstage app
npx @backstage/create-app@latest
The above will prompt you to name your backstage project.
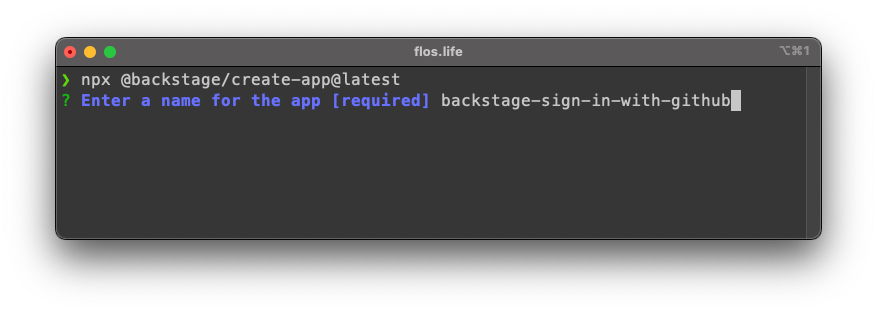
After that, the project will be scaffolded. We can cd into it and run it using yarn.
# cd into your project directory
cd backstage-sign-in-with-github
# i needed to do this, in the future you probably wont
yarn add --cwd packages/backend @swc/types
# start in dev mode
yarn dev
adding github authentication
creating a github oauth app
We will need to create a github oauth app to use with the login, to do so, navigate to: https://github.com/settings/applications/new
(Thats settings -> developer settings -> OAuth Apps)
Give your app a name, any homepage url and callback url:
http://localhost:7007/api/auth/github
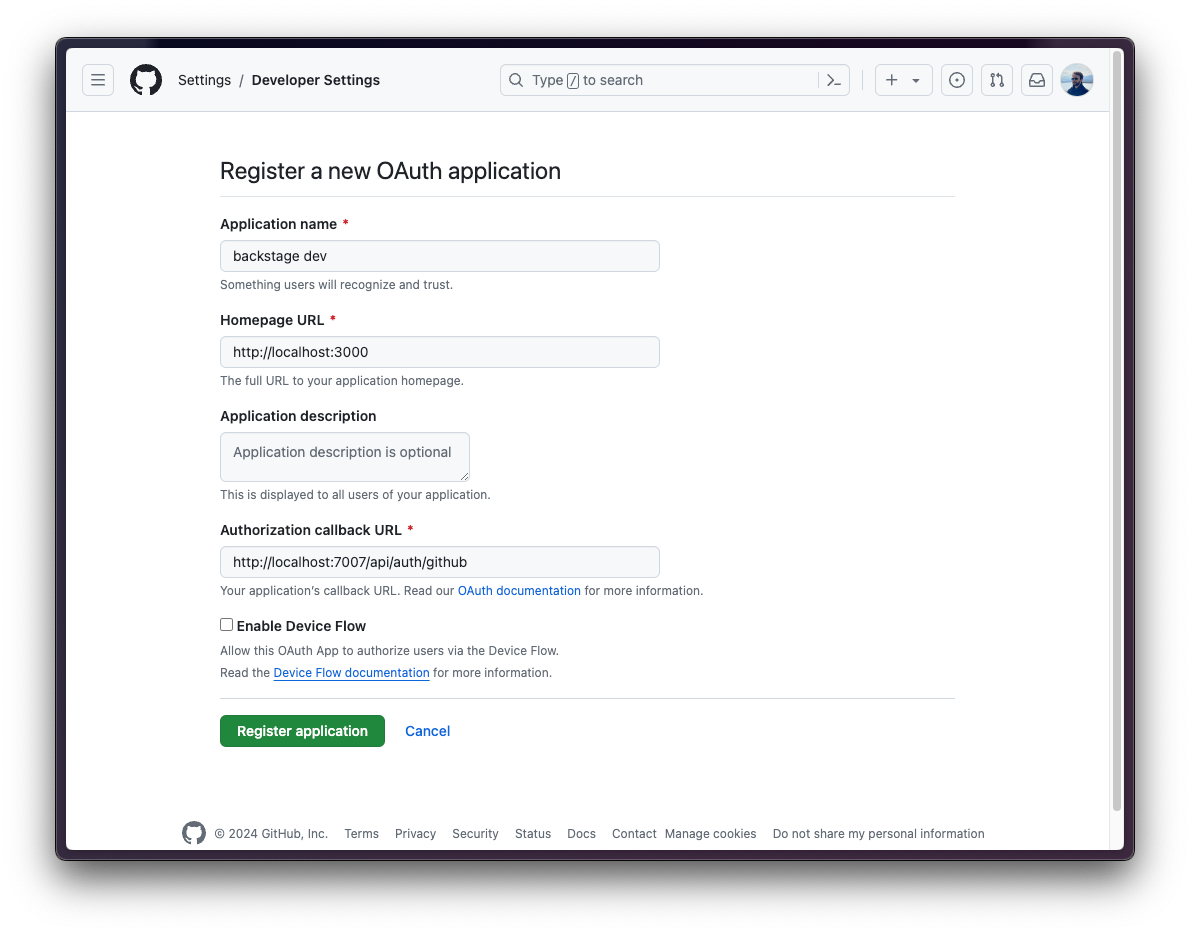
After registering the application, you will receive a client id and the can generate a new client secret. We will need both in the next step.
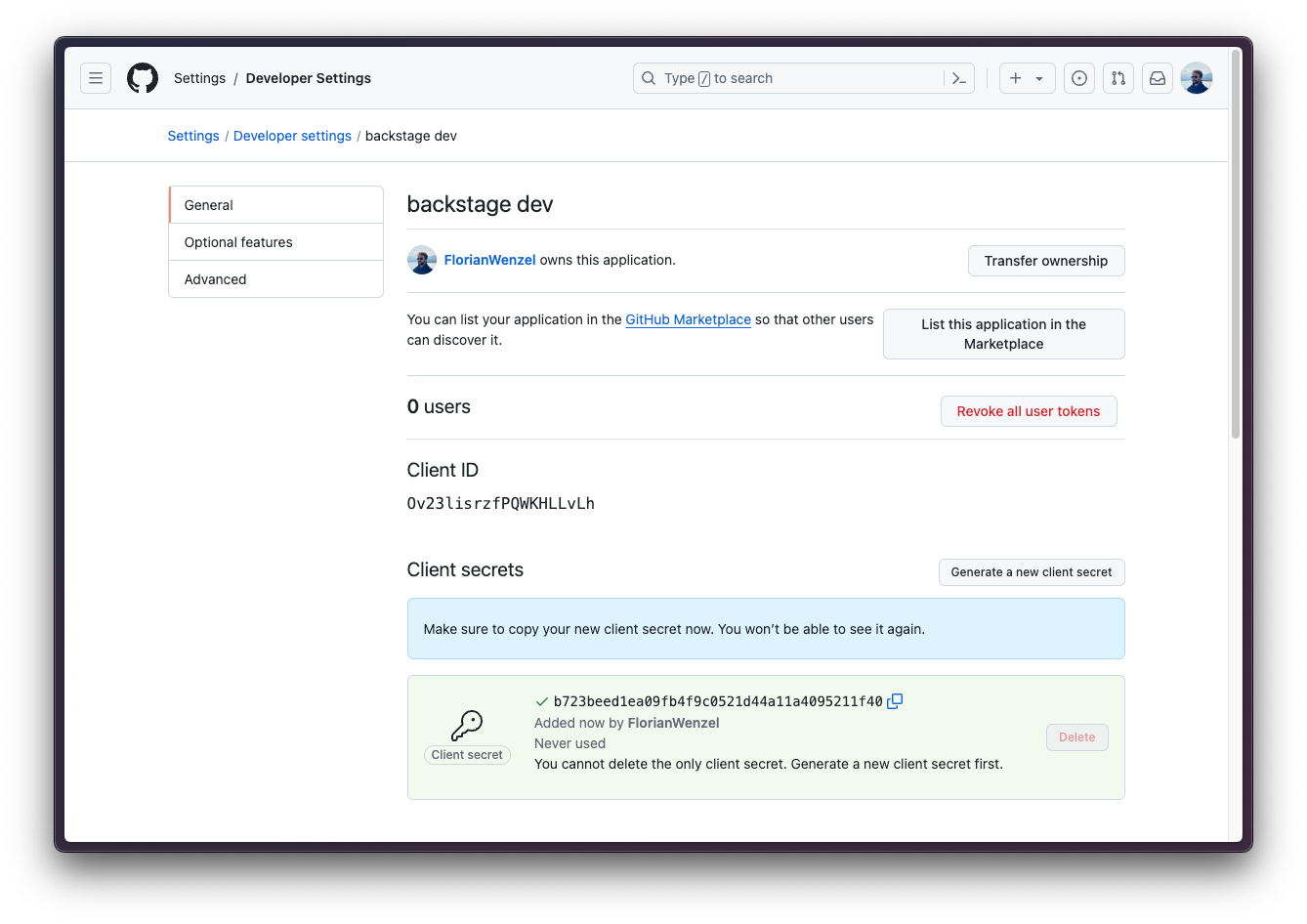
add github auth provider to backstage backend
The in the step above generated client id and client secret we will use inside the app-config.yml in the project root:
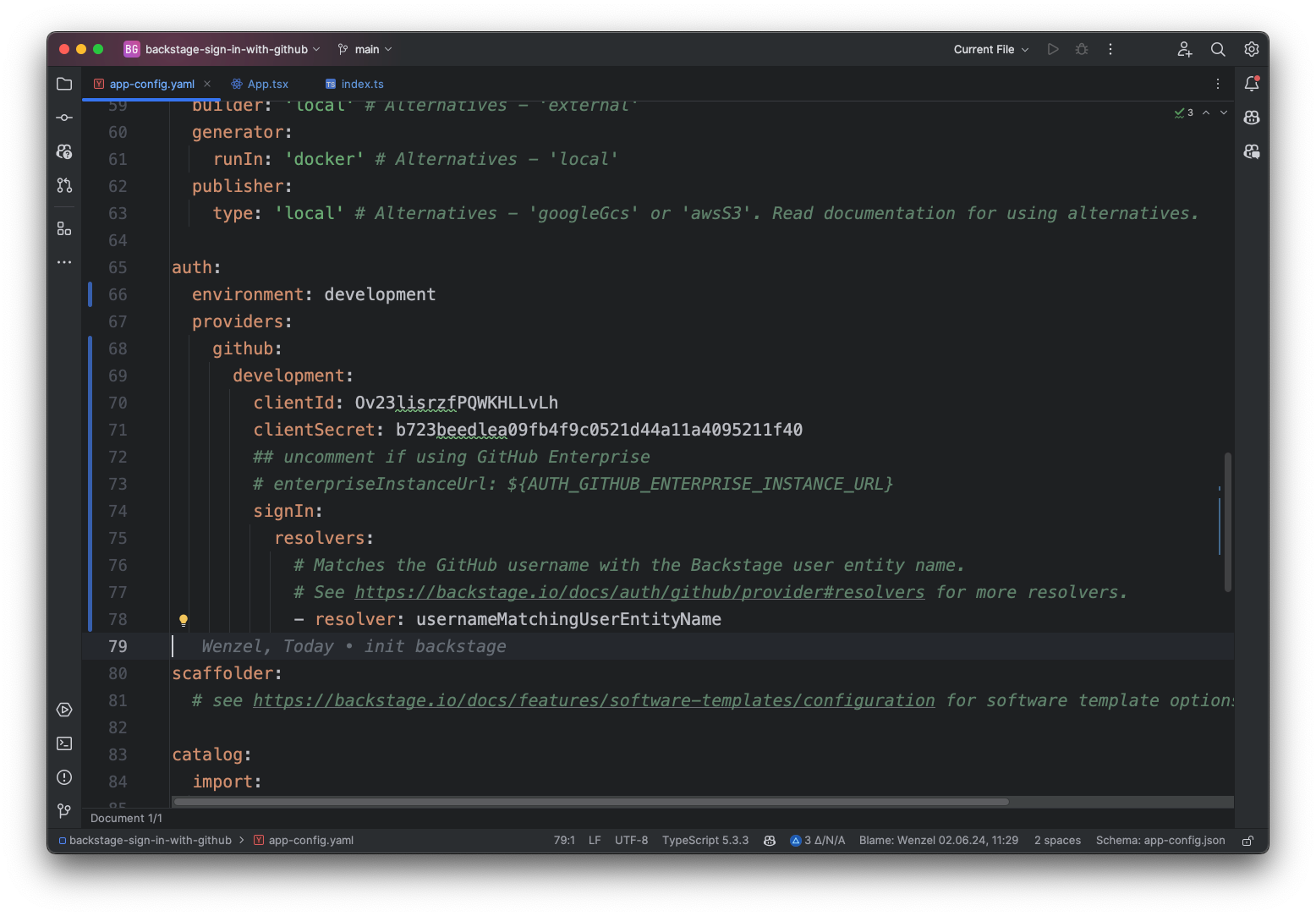
auth:
environment: development
providers:
github:
development:
clientId: Ov23lisrzfPQWKHLLvLh
clientSecret: b723beedlea09fb4f9c0521d44a11a4095211f40
signIn:
resolvers:
- resolver: usernameMatchingUserEntityName
This tells backstage we want to use the github provider, at least when backstage is launched in development mode.
There are multiple resolvers available:
- usernameMatchingUserEntityName: matches the username of the github account with an in backstage registered account
- emailLocalPartMatchingUserEntityName: matches everything left of the @ from the email address with a username registered in backstage
- emailMatchingUserEntityProfileEmail: matches the github accounts email with the backstage accounts email
I choose the usernameMatchingUserEntityName resolver. In the default app-config.yaml file, the guest provider is enabled, you can keep it enabled if you want to.
We also need to import the github auth provider module like this in packages/backend/src/index.ts
backend.add(import('@backstage/plugin-auth-backend-module-github-provider'));
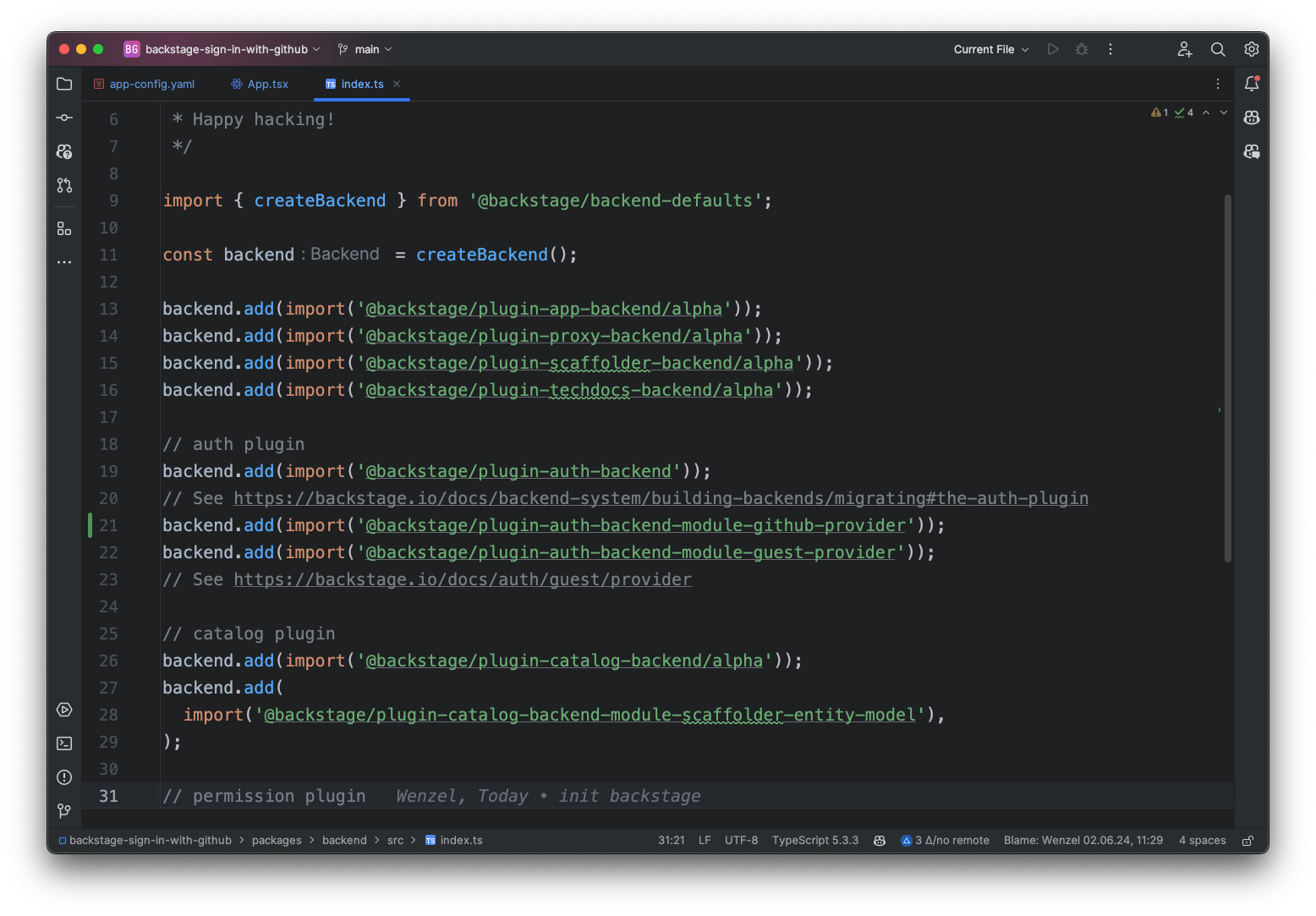
add auth provider to frontend
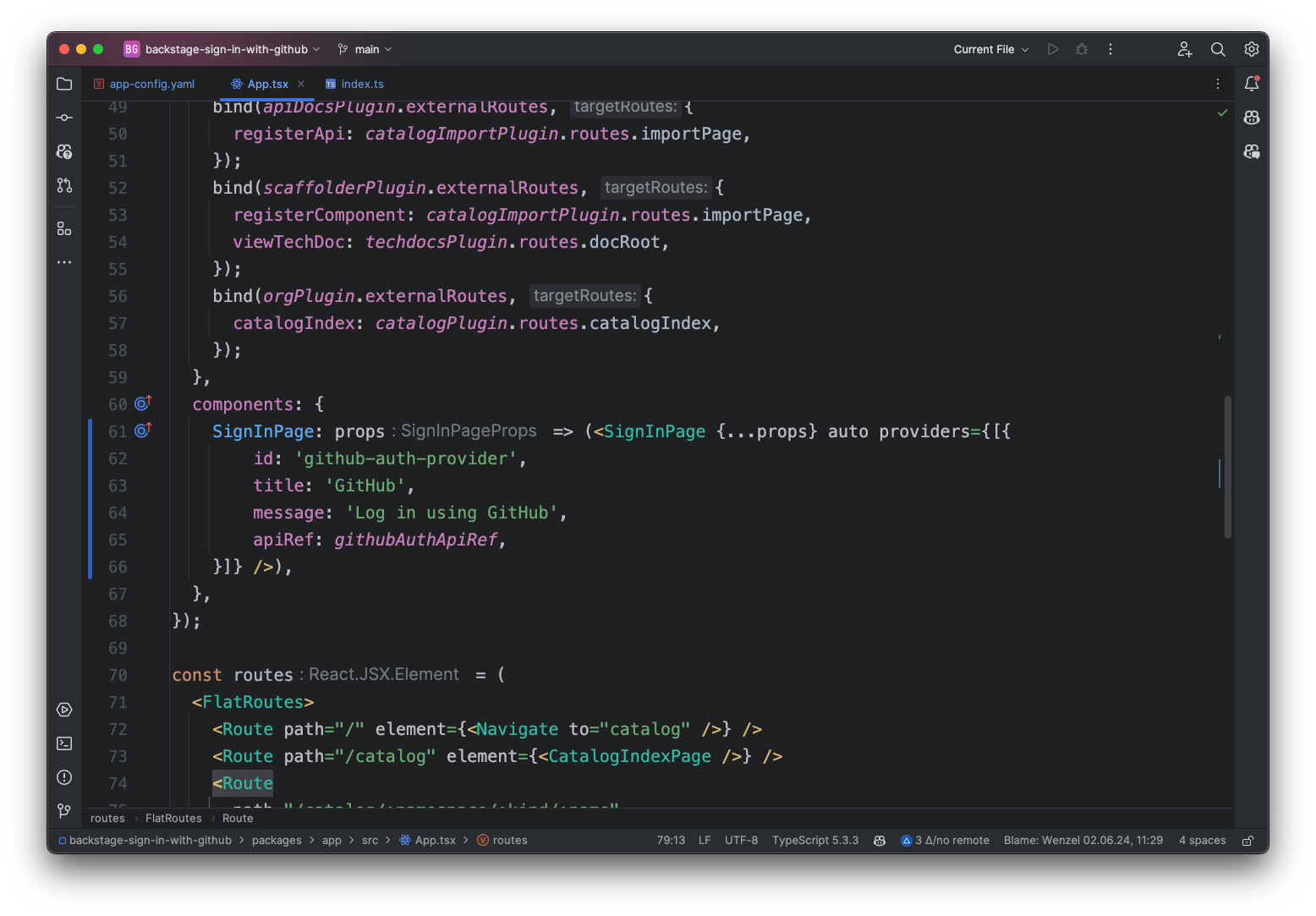
In the frontend we need to add the provider to the SignInPage, see the screenshot above. Per default it comes with the "guest" provider, you can leave that on if you want to.
import { githubAuthApiRef } from '@backstage/core-plugin-api';
components: {
SignInPage: props => (<SignInPage {...props} auto providers={[{
id: 'github-auth-provider',
title: 'GitHub',
message: 'Log in using GitHub',
apiRef: githubAuthApiRef,
}]} />),
},
adding a user to backstage
The login is done! But there is no user matching your github account yet. In practice, you probably want to use the github org data plugin, but to get started, we can add it another way.
Per default, backstages comes with an org.yaml in the examples directory in the project root. In there there already is one user and one group defined. We can add our user to it. Since i am using name matching, i need to add my github username as a user.
---
apiVersion: backstage.io/v1alpha1
kind: User
metadata:
name: florianwenzel
spec:
memberOf: [guests]
---
Now we can sign into backstage using github! 🥳